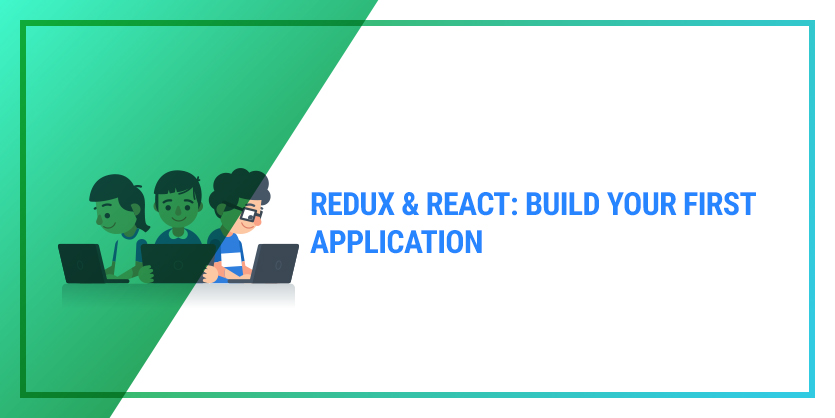
Redux is not related to React anyhow. Users interact with programs. They move through the data sections, search, filter, select, add, update and delete them. When the user interacts with the UI, the program state changes, and these changes are displayed to the user in the UI. The screens and menu bars appear and disappear. The visible content changes. Various indicators switch on and off. In React, the user interface reflects the state of the application.
The state can be expressed in framework components with a single JavaScript object. At the moment of changing the state, the component displays a new user interface showing these changes. What can be more functional? When retrieving data, the React component displays them in the form of a UI. Based on their changes, this framework will update the interface to reflect these changes in the most rational way.
To manage the state and reduce the amount of template code, you can apply Redux. Redux provides updates of the state as responses to actions. We’ll show you how to develop a light todo program.
How to install React?
Use this line to install React bindings or get the latest builds that will export window.ReactRedux global with the <script> tag.
Two Redux components
Keep in mind that two components are separated. What is the major difference between them? Presentational elements are in charge of styles, markups or and everything else related to the appearance. They are not aware of Redux or how the data is loaded in the program. Container elements are in charge of the way things work in the program.
They give information and behaviour to other components. Such a separation allows developers to reuse them in other projects. Designers can play with the presentational elements to create a perfect UI without any risk to change the logic.
It’s no good to write container elements manually as React Redux runs a lot of optimizations regarding performance. We advise using connect(). We’ll demonstrate its use in the code samples below.
How to build the structure?
Start building the structure for the UI after you finish designing the structure of a root state object. The design description will be short enough. Imagine a checklist of to-do actions on the screen. You cross out one action with a tap. There’s a special field where you can add a new item to the list. And there will be a button to show you everything, completed or uncompleted items.
How to build presentational components?
Here they are with the props:
These components render the look without indicating the data sources or any information on how we can make updates. You will have these elements the same after migrating from Redux to anything else thanks to the absence of the dependency on Redux.
How to build container components
Let’s take container elements for uniting Redux and presentational elements. We’ll take VisibleTodoList container for the presentational one that will send requests to the Redux store to find out the instruction to enable the visuals filter. We’ll require a FilterLink container to provide updates to the filter. This container will render Link that is responsible for rendering the right action after a tap:
How to build other components?
You may face some situations when it’s complicated to define the type of a container. Let’s take a look at the example below where function and form are united:
It’s not the time for dividing it into two elements. Wait until a tiny element containing presentation and logic grows to see how it can be divided.
How to implement components?
Presentational elements are easy to start with and there’s no need to dive into the bindings theory.
There’s no need to analyze these framework elements. We’ll write functional elements that are stateless. This doesn’t imply turning presentational components into functions.
components/Todo.js
components/TodoList.js
components/Link.js
components/Footer.js
connect() function is responsible for setting up container elements. store.subscribe() is used for viewing Redux state tree. mapStateToProps is a function that allows using connect(). Let’s take a look at the sample. We define a filtering function for state.todos with the help of state.visibilityFilter:
Container components have one more feature. They dispatch actions. Determine mapDispatchToPros() function to get dispatch() method and give you back the props for inserting into a presentational component. Take a look at the example where we have onTodoClick prop that will be inserted into TodoList element by VisibleTodoList. And TOGGLE_TODO action will be dispatched by onTodoClick:
We call connect() and pass the two functions to make VisibleTodoList:
You’ve seen the essentials of the React Redux API. Review information about the computing derived data if you feel worried that there’s a too big number of new objects made by mapStateToProps. You will find all the other components below. Scroll down to see them all.
container/FilterLink.js
containers/VisibleTodoList.js
containers/AddTodo.js
Logic and appearance for the AddTodo element are put together:
Uniting containers
components/App.js
How to deal with the Store?
Container elements cannot subscribe to the Redux Store if they don’t have access to it. There’s one way out. You can send it as if it was a prop to every single element. However, you might get exhausted as there’s a need to write store even via presentational elements as they’re rendering containers somewhere deep in a tree.
Use a React Redux element that gives access to the store for all container elements inside the program. You run it only one time while the root element is rendered:
index.js
Perhaps, Flux is some kind of revelation for the frontend. For a backend, the difference between CQRS and Flux is not great. React is what we need. It can depend on props (read-only) or state (mutable). Depending on the state and props, the component can be displayed in different ways.
This logic is contained in the render method. The component does not reproduce itself. Instead, it relies on the React ecosystem. We can either change our state (using the setState method) or be reproduced from the outside (new props are transferred). Event handlers for UI elements are passed through props.
The state of the application is stored in Redux and is a JSON object. Redux monitors the change of the object. Change is considered to adjust the reference, so instead of changing the ongoing state, you should design a new one by copying and modifying the old one. The easiest way to do this is through a spread operator.
The react-redux package implements the redux state => react component one-way by using the connect method. When the state changes, Redux itself reproduces the necessary components, passing props to the dispatch and a part of the general state. It’s up to you which part of the state and which functions on the basis of store.dispatch to transmit. I recommend passing all component event handlers and don’t display the dispatch in the components.
When it comes to choosing tools for solving problems in React projects, it all comes down to the main thing – optimality. Each product requires a special approach. If we are talking about the unlimited budget but a limited time frame, this is one approach. If the situation is completely opposite, it requires a choice of other technologies. As a rule, we are faced with the fact that both the budget and the time are limited. In this case, the most successful (optimal) choice of tools will allow us to get the most qualitative result.